Graphics Rendering
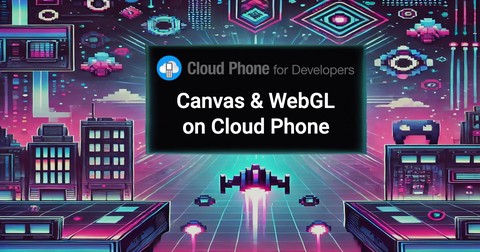
Web Graphics
Cloud Phone brings sophisticated graphics capabilities to budget feature phones. Develop interactive 2D and 3D widgets using Canvas and WebGL.
The Canvas API
Understanding the Basics
The Canvas API is a powerful yet straightforward way to draw 2D graphics using JavaScript. A Canvas is a bitmap surface to draw shapes, images, and text. The rendering context, obtained through getContext('2d')
, serves as the primary interface for drawing operations.
const canvas = document.createElement('canvas');
const ctx = canvas.getContext('2d');
// Setting canvas dimensions (QVGA)
canvas.width = 240;
canvas.height = 320;
// Basic drawing example
ctx.fillStyle = 'blue';
ctx.fillRect(10, 10, 100, 100);
Performance
Restrict canvas dimensions to the screen size. Canvas works well with frequent updates to the entire drawing surface, but its best to limit canvas dimensions to match the screen size. The Canvas API operates on an immediate mode rendering model, meaning each frame must be redrawn entirely, even if only a small portion changes. Drawing a surface larger than the screen, then downscaling, can unnecessarily hurt performance.
Security
A canvas becomes tainted when you draw any data that was loaded from another origin without Cross-Origin Resource Sharing (CORS) approval. A tainted canvas is no longer considered secure, and any attempts to retrieve image data back from the canvas–via getImageData()
, toBlob()
, toDataURL()
or captureStream()
–will throw a SecurityError
. Ensure the Access-Control-Allow-Origin
is configured permit cross-origin access to image files with the *
wildcard, or to the requesting origin if credentials are required.
WebGL: 3D Graphics
WebGL brings hardware-accelerated 3D graphics to Cloud Phone through a standard JavaScript binding to OpenGL ES. OpenGL can rendering sophisticated 3D applications and games directly on feature phones. WebGL operates at a lower level than Canvas, requiring an understanding of graphics programming concepts like vertices, shaders, and the graphics pipeline.
const canvas = document.createElement('canvas');
const gl = canvas.getContext('webgl');
// Initialize WebGL context
if (!gl) {
console.error('WebGL not supported');
return;
}
// Basic WebGL initialization
gl.clearColor(0.0, 0.0, 0.0, 1.0);
gl.clear(gl.COLOR_BUFFER_BIT);
Shaders and the Graphics Pipeline
WebGL relies on shader programs written in GLSL (OpenGL Shading Language) to define how vertices and pixels are processed. A typical WebGL application requires at minimum a vertex shader for positioning geometry and a fragment shader for determining pixel colors.
// Vertex Shader
attribute vec4 aVertexPosition;
uniform mat4 uModelViewMatrix;
uniform mat4 uProjectionMatrix;
void main() {
gl_Position = uProjectionMatrix * uModelViewMatrix * aVertexPosition;
}
// Fragment Shader
precision mediump float;
void main() {
gl_FragColor = vec4(1.0, 1.0, 1.0, 1.0);
}
WebGL Fundamentals offers many resources to learn more about shaders, image processing, lighting, transforms, textures, and more.
WebGPU
WebGL is not supported on Cloud Phone at this time.
WebGPU is an advanced, lower-level technology to access GPU capabilities with a more modern, efficient API compared to WebGL. Cloud Phone servers run Chromium on Linux where WebGPU is only experimentally available behind the #enable-unsafe-webgpu
and #enable-vulkan
feature flags.
Choosing the Right Technology
Canvas 2D
- Simple 2D graphics and visualizations
- Pixel manipulation
- Basic gaming applications
WebGL
- 3D graphics and games
- Complex visualizations requiring hardware acceleration
- Applications needing maximum performance
- Custom shader effects and advanced rendering techniques
WebGPU
- Not available on Cloud Phone
- Next-generation graphics applications
- Computing applications requiring GPU acceleration
Best Practices
Performance Optimization
Graphics performance significantly impacts user experience. Use requestAnimationFrame
loops for smooth animations and batch drawing operations to reduce redraws.
// Efficient animation loop
function render(timestamp) {
// Clear and update canvas
ctx.clearRect(0, 0, canvas.width, canvas.height);
// Perform drawing operations
updateAndDraw();
// Schedule next frame
requestAnimationFrame(render);
}
requestAnimationFrame(render);
Frame Rate
Cloud Phone apps run at a variable frame rate up to 20 frames per second (FPS) on stable 4G or WiFi networks with strong signal strength. Interactive puzzle, arcade, trivia, strategy, board, and card games all work well on T9 devices. However, due to the variable frame rate, real-time games that require consistent response times are not well suited for Cloud Phone.
Conclusion
Web graphics technologies continue to evolve. Cloud Phone can run most apps and games that use Canvas or WebGL to render 2D and 3D scenes. D-pad navigation is the perfect choice for classic arcade and puzzle games.