Privacy & Security
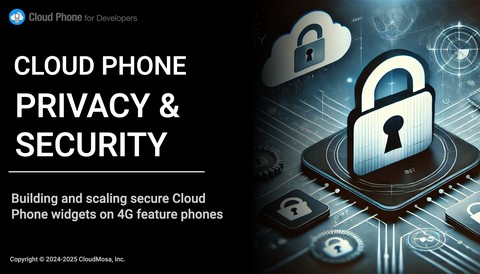
Cloud Phone provides a secure platform to access information, store data, and render web apps on feature phones. While CloudMosa manages security of Cloud Phone, security in Cloud Phone is your responsibility. You are responsible for protecting the confidentiality and integrity of your data and the privacy of your users.
This article covers basic technologies and principles to secure your Cloud Phone widget.
Technologies & APIs
HTTPS
Cloud Phone requires all connections be secure using the encrypted Hypertext Transfer Protocol Secure (HTTPS). Connections over insecure HTTP are not allowed and will be blocked.
HSTS
Use the Strict-Transport-Security
header to enable HTTP Strict Transport Security (HSTS) to inform browsers that your site should only be accessed using HTTPS. This ensures that future attempts to access your site using HTTP should automatically be upgraded to HTTPS.
HSTS is recommended over the Upgrade-Insecure-Requests
header, which does not ensure that users visiting your site via links on third-party sites will be upgraded to HTTPS for the top-level navigation.
Network Security
Add the CloudMosa data center IP addresses ranges to your allowlist to ensure Cloud Phone user traffic does not get rate limited or misidentified as bot traffic.
External Content
Sanitize all user input and external content to remove unsafe characters and potentially harmful code from forms, files, HTML, and more.
Always sanitize user input on your server. Malicious actors can circumvent client-side validaion by manually initiating API calls or intercepting and modifying outgoing requests.
Cross-Site Scripting (XSS)
Cross-Site Scripting vulnerabilities are common when users enter data that gets reflected or stored by your server and subsequently rendered as HTML, or set unsafely as HTML content, attributes, or in URI schemes.
Trusted Types
Use the Trusted Types API to sanitize HTML strings before rendering using risky injection sinks like innerHTML
.
const escapeHTMLPolicy = trustedTypes.createPolicy("myEscapePolicy", {
createHTML: (string) => string.replace(/&/g, "&").replace(/</g, "<").replace(/"/g, """).replace(/'/g, "'")
});
let el = document.getElementById("myFigure");
const escaped = escapeHTMLPolicy.createHTML("<img src=x onerror=alert(1)>");
el.innerHTML = escaped; // <img src=x onerror=alert(1)>
The policy named default
is used wherever a string is provided to a sink that requires a Trusted Type. Some sanitization libraries like DOMPurify support Trusted Types and can return a TrustedHTML
object.
SQL Injection
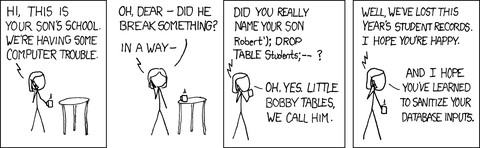
SQL injection is one of the most common web hacking techniques. Attackers will provide SQL commands as input in an attempt to execute commands against your database.
Use an Object Relational Mapper (ORM), or prepared statements with paramaterized queries to include potentially-unsafe user input in database operations. Avoid dynamic query generation using escaped strings as this can fail unexpectedly.
Web Crypto
Cloud Phone offers cryptographic primitives via the Web Crypto API. For instance, use Web Crypto to generate random Universally Unique Identifiers (UUID).
UUID
let uuid = crypto.randomUUID();
console.log(uuid);
// "3c4e741da-0ffc-4e5b-9491-b69d2744b590"
Hash
Use crypto.subtle.digest
to generate hashes (digests). Hashes are one-way functions that consistently transform the input into a universally unique output.
const passwordPin = '123456';
function bufferToHex(buffer) {
return Array.from(new Uint8Array(buffer))
.map((b) => b.toString(16).padStart(2, '0'))
.join('');
}
const encoder = new TextEncoder();
(async () => {
const hashBuffer = await crypto.subtle.digest(
'SHA-256', // Algorithm
encoder.encode(passwordPin)
);
console.log(bufferToHex(hashBuffer));
// 8d969eef6ecad3c29a3a629280e686cf0c3f5d5a86aff3ca12020c923adc6c92
...
})();
The Web Crypto API can also be used for encryption and decryption, signing & verification, and more. It’s easy to use the Web Crypto API incorrectly in ways that are insecure and unreliable. Prefer well-tested libraries and frameworks, rather than writing your own cryptographic applications from scratch.
Same Origin Policy (SOP)
All Cloud Phone widgets are subject to the Same Origin Policy (SOP). See Networking for information on the Same Origin Policy and Cross-Origin Resource Sharing for more information.
Content Security Policy (CSP)
A Content Security Policy (CSP) allows you to restrict certain behaviors for increased security. A CSP can disallow inline scripts or eval
, both of which reduce the attack surface for Cross-Site Scripting (XSS) attacks.
Cloud Phone respects CSPs just like Google Chrome. Read to learn more and check out Google’s CSP Evaluator to identify subtle CSP bypasses.
Catch policy violations by listening for the securitypolicyviolation
event.
document.addEventListener("securitypolicyviolation", (e) => {
console.log(e.blockedURI);
console.log(e.violatedDirective);
console.log(e.originalPolicy);
});
Alternatively, use a ReportingObserver
to access security policy violations as well as deprecation and intervention warnings.
const observer = new ReportingObserver(
(reports, observer) => {
for (const report of reports) {
console.log(report.type, report.url, report.body);
}
},
{ buffered: true }
);
observer.observe();
The option buffered: true
allows you to see reports generated before the reporter was created.
Finally, you can also receive violations by providing a report URI using the report-to
directive with the Content-Security-Policy
and Content-Security-Policy-Report-Only
response headers.
Content-Security-Policy: …; report-uri https://endpoint.example.com; report-to endpoint_name
Eval
Although eval
is permitted by default on Cloud Phone, it’s stronngly recommended that you never use direct eval()
.
eval('console.log("hello from eval()")');
const sum = new Function("a", "b", "return a + b");
setTimeout("console.log('Hello from setTimeout')", 1);
Subresource Integrity (SRI)
Subresource Integrity (SRI) allows Cloud Phone to verify that remote resources like CSS and JS are delivered without manipulation. This is achieved by providing a cryptographic hash that a fetched resource is matched against.
Set the integrity
attribute on a <script>
or <link>
. Then when Cloud Phone fetches the resource, it will first be compared against the expected hash given in the integrity
value.
<script src="https://example.com/example-framework.js" integrity="sha384-oqVuAfXRKap7fdgcCY5uykM6+R9GqQ8K/uxy9rx7HNQlGYl1kPzQho1wx4JwY8wC" crossorigin="anonymous"></script>
SRIHash.org offers a free SHA384 hash generator, or implement SRI in your build process.
Data Storage
All Cloud Phone widget data is stored encrypted on Cloud Phone servers. Private keys are generated and stored on the user’s phone, used but never stored on Cloud Phone servers. See Data Storage for more information on supported APIs and storage quotas.
Cache
Cloud Phone only caches responses for the duration of a session. Caches are not persisted or shared between users or across sessions.
Code Obfuscation
javascript-obfuscator and similar tools provide code obfuscation. Although not a strong security practice, obfuscation increases effort required of skilled attackers by making it more difficult to reverse-engineer web applications through dead code injection, string encryption, and control flow flattening.
WebAssembly
Sandbox
WebAssembly (Wasm) code runs in a sandboxed environment. Cloud Phone uses the same V8 WebAssembly runtime as Chromium. The Wasm runtime on Cloud Phone is compatible with the corresponding version of Google Chrome.
Reverse Engineering
WebAssembly can be used to create code that is difficult to understand or reverse engineer. The WebAssembly binary format is highly optimized, discarding a significant amount of information and constructs from the source language like Java, Rust, or Go. WebAssembly disassemblers and decompilers are limited, making Wasm a compilation target for intellectual property (IP) protection.
Code Injection
Because of its computational performance and obfuscated binary format, Wasm is a common target for code injection threats. Typically, a Wasm module is loaded to perform profit-generating activities like cryptocurrency mining in the browser. Only instantiate well-known and documented Wasm binaries, and avoid introducing unnecessary dependencies to minimize supply chain risks.
Best Practices
- Use HTTPS – Always use HTTPS, its required for all Cloud Phone widhets
- Use a Firewall – Restrict access to your app to CloudMosa data centers
- Validate Inputs – Clean user inputs to prevent harmful code introduction
- Sanitize Outputs – Escape dangerous characters before showing user data
- Keep Software Updated – Update your libraries and dependencies
- Use Secure Cookies – Set cookies with
Secure
andHttpOnly
flags - Protect Against CSRF – Use CSRF tokens to prevent unauthorized actions
- Escape Special Characters – Prevent XSS by escaping user input
- Set a CSP – Restrict what scripts and resources your site can load
- Use Parameterized Queries – Avoid SQL injection with prepared statements
- Monitor for Security Issues – Regularly check logs for suspicious activity
- Handle Errors Properly – Don’t reveal system details in error messages
- Disable Unused Features – Remove unnecessary services and APIs
- Use Strong Authentication – Require strong passwords and support 2FA
- Limit Logins – Block repeated failed logins to prevent brute-force attacks
Conclusion
Securing web applications is a complex and ever-evolving task, and Cloud Phone widgets are no exception. By following a set of best practices, you can design secure and reliable apps that users can enjoy around the world.
Contact Us
If you believe you have discovered a vulnerability in Cloud Phone, or have a security incident to report, please contact us.
Get Support