Data Storage
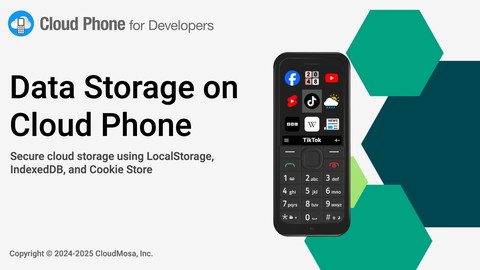
Cloud Phone runs on devices like the MTR Speed with as little as 16 MB of storage. Modern apps require more than 16 MB of data storage to remember session cookies, settings & preferences, and perists data against unreliable network conditions. To accomplish this, Cloud Phone provides standard Web APIs to read and write data securely on cloud servers.
Secure Cloud Storage
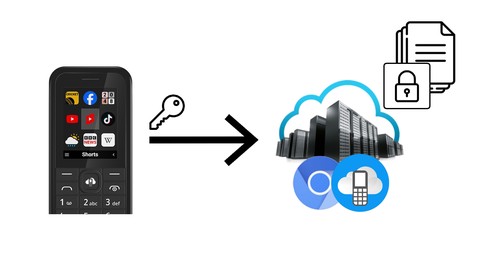
Data is encrypted using a unique private key stored only on the device. The key is sent to Cloud Phone servers when the app is launched. This allows Cloud Phone to securely store more data than the device has in available storage, and reduces the latency for reading and writing data.
At a Glance
- Cloud Phone supports LocalStorage, IndexedDB, and Cookie Store
- Data is stored securely on Cloud Phone servers
- Users can “Clear Data” at any time
LocalStorage
LocalStorage persistently stores UTF-16 strings as key-value pairs up to a 5 MB limit. LocalStorage on Cloud Phone works the same as on desktop.
localStorage.setItem("catName", "Lovie");
let catName = localStorage.getName("catName");
Call clear
to remove all stored keys.
localStorage.clear();
Use the storage
event to synchronized frames within a page. You cannot open a popup window since Cloud Phone is a single-tab browser.
window.addEventListener('storage', (event) => {
if (event.key === 'catName') {
renameCat(event.newValue);
}
});
SessionStorage
SessionStorage is similar to LocalStorage except that data is automatically cleared when the user exits the Cloud Phone app. Unlike volatile memory, data is persisted between reloads. A common use case for SessionStorage is to track writing changes and restore a text field in case the user refreshes the page.
if (localStorage.getItem("shouldAutosave") === "true") {
// ...
}
function onEdit() {
localStorage.setItem("shouldAutosave", "true");
}
Cloud Phone does not have a refresh button. Call location.reload()
to manually reload your app.
IndexedDB
IndexedDB is a lower-level, asynchronous API for storing structured and binary data. IndexedDB has no fixed quota, but individual blobs cannot exceed 50 MB.
IndexedDB is accessed via named databases.
const request = indexedDB.open("TestDatabase");
request.onsuccess = (event) => {
let database = event.target.result; // IDBDatabase
};
Databases have numbered versions that trigger an upgrade request on first access, or by specifying a number higher than the previous version. Objects within a database are stored in object stores. Object stores can optionally have a key generator for ordered sequences and a key path for indexed queries.
request.onupgradeneeded = (event) => {
const database = event.target.result;
const noteStore = database.createObjectStore("notes",
{ keyPath: "id", autoIncrement: true }
);
};
Object stores are read or written using transactions.
let transaction = database.transaction(
database.objectStoreNames, IDBTransaction.READ_WRITE
);
transaction.oncomplete = function(event) {
console.log("All done!");
};
transaction.onerror = function(event) {
console.error(event);
};
const noteStore = transaction.objectStore("notes");
//use put versus add to always write, even if exists
request = noteStore.add({
title: "Groceries",
body: "Milk, Eggs, Rice...",
});
add()
inserts a new record, or triggers a ConstraintError
error if one already exists. put()
is an UPSERT that updates an existing record, or inserts a new one if none exist.
Although IndexedDB does not have a fixed quota, a QuotaExceededError
is thrown when your app has stored too much data.
Persistent and Unlimited Storage
Persistent Storage and the unlimitedStorage
permissions are not available on Cloud Phone.
navigator.storage.persist()
always returns false
.
navigator.storage.estimate()
reports a quota
value much higher than what your app is allowed to store.
Cookies
Cloud Phone perists cookies created using the Set-Cookie
header, document.cookie
, or Cookie Store API. cookieStore
is the easiest way to access cookies in JavaScript.
const day = (24 * 60 * 60 * 1000);
cookieStore
.set({
name: "NeedsBreak",
value: "true",
expires: Date.now() + day,
domain: location.hostname,
})
.then(() => console.log("It worked!"))
.catch((e) => console.error(e));
Cloud Phone adheres to the same cookie policies as Google Chrome. For instance, cookies specified with HttpOnly
attribute cannot be accessed using JavaScript. Third-party cookies are also restricted via the SameSite
attribute.
Listen for changes or deleted cookies with the change
event on cookieStore
.
cookieStore.addEventListener("change", (event) => {
let needsBreak = event.changed
.find((cookie) => (cookie.name === 'NeedsBreak'));
if (needsBreak) {
// Tell user to come back after needsBreak.expired
}
})
Clear Data
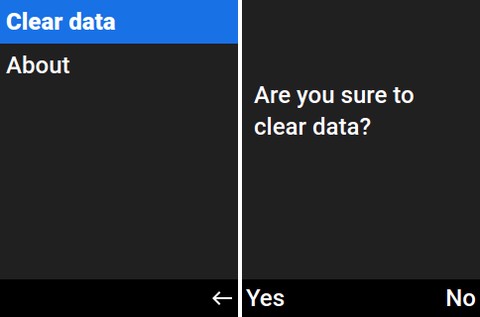
Users can Clear Data at any time from the settings menu on the Cloud Phone homescreen. Once confirmed, all data on the Cloud Phone app and server is purged. This includes all data stored in LocalStorage, IndexedDB, and Cookies.
Conclusion
Cloud Phone supports most common data storage APIs including LocalStorage, IndexedDB, and Cookie Store. All other data is cleared when the user exists the Cloud Phone app. For critical information, authenticate users and backup data to their account.